This guide showcases the necessary steps to implement automated issuing of invoices on behalf of merchants for marketplace transactions using Space Invoices API.
Onboard a merchant
The first step of the process is on-boarding a new or existing merchant to invoicing by Creating an Organization and optionally Creating an Account for them.
The organization represents the merchant's business or other type of entity, and will house all the invoices created on their behalf.
The account represents the merchant's user and will allow us to limit their access only to their organization and invoices.
Note: By using your root Space Invoices account's access token for API calls, you are granted access to all resources created by you or by any of your child accounts.
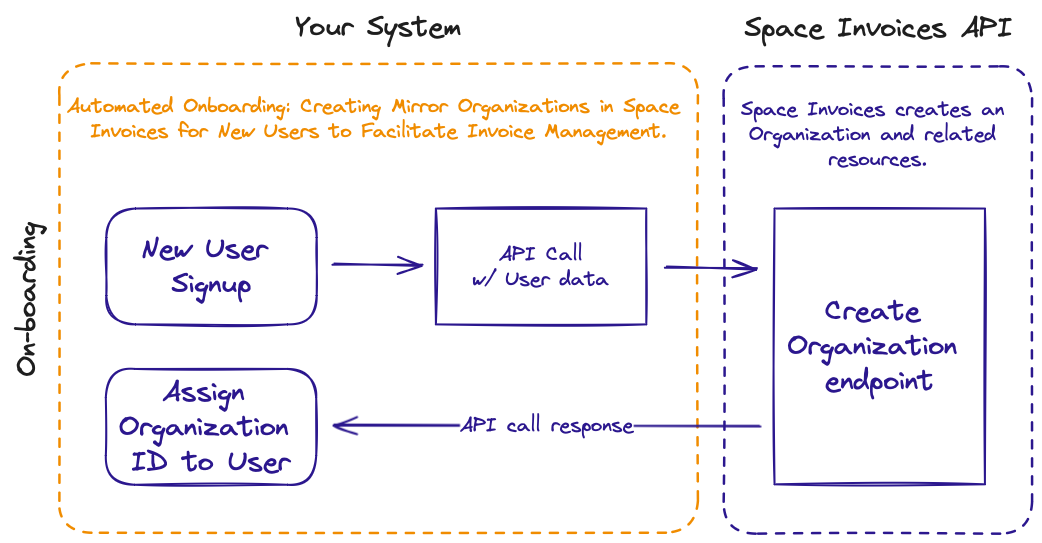
Creating an Organization
We start by creating an Organization representing the merchant’s business.
An organization can represent a business or other entity that issues invoices and other documents.
An organization can be created using the Create Organization API endpoint.
Note: Besides creating the organization itself, the API also auto-generates some additional content based on the country and language of the organization, including adding tax rates, default email and document messages, and currency.
The “Creating an Account” and “Link the created Account and Organization” steps are optional, and are only required in case of granting access to the invoicing UI to merchants.
Don't need an Account for your merchant? Skip to Creating and sending an Invoice section >
const response = await fetch('https://api.spaceinvoices.com/v1/Accounts/{id}/Organizations', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"name": "Example Merchant co.",
"address": "Example street 1",
"city": "Brussels",
"zip": "10000",
"country": "Belgium"
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/Accounts/{id}/Organizations' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"name": "Example Merchant co.",
"address": "Example street 1",
"city": "Brussels",
"zip": "10000",
"country": "Belgium"
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/Accounts/{id}/Organizations"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'name': 'Example Merchant co.',
'address': 'Example street 1',
'city': 'Brussels',
'zip': '10000',
'country': 'Belgium'
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"name": "Example Merchant co.",
"address": "Example street 1",
"city": "Brussels",
"zip": "10000",
"country": "Belgium",
"countryAlpha2Code": "BE",
"locale": "nl-BE",
"active": true,
"activatedAt": "2023-04-28T19:41:00.914Z",
"createdAt": "2023-04-28T19:41:00.930Z",
"updatedAt": "2023-04-28T19:41:00.930Z",
"custom": {},
"_data": []
}
Create an Account (optional)
Secondly, we create an Account for the merchant, this will provide the option to grant scoped access to the merchant’s data.
An account can be created using the Create Account API endpoint.
Note: A placeholder or bogus email can be used instead of the actual merchant’s email as well.
const response = await fetch('https://api.spaceinvoices.com/v1/Accounts', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"email": "EMAIL",
"password": "PASSWORD"
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/Accounts' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"email": "EMAIL",
"password": "PASSWORD"
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/Accounts"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'email': 'EMAIL',
'password': 'PASSWORD'
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"email": "EMAIL",
"createdAt": "2023-04-28T19:41:00.930Z",
"updatedAt": "2023-04-28T19:41:00.930Z",
"custom": {},
"_data": []
}
Linking the created Account and Organization (optional)
Lastly, to finish the on-boarding, the created account and organization should be linked together, this will grant the created account access rights to the organization.
To link (invite) an account to organization the link API call can be used.
Setting requireConfirmation: false
will automatically add the account to the organization without requiring them to confirm.
Setting sendEmail: false
will skip sending an email notification to the account's email.
const response = await fetch('https://api.spaceinvoices.com/v1/Organizations/{id}/invite', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"accountId": "ACCOUNT_ID",
"requireConfirmation": false,
"sendEmail": false
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/Organizations/{id}/invite' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"accountId": "ACCOUNT_ID",
"requireConfirmation": false,
"sendEmail": false
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/Organizations/{id}/invite"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'accountId': 'ACCOUNT_ID',
'requireConfirmation': false,
'sendEmail': false
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Create and Send an Invoice
Creating an invoice is as simple as writing a few lines of code, requiring only the most basic data by default. The API automatically fills in the remaining details based on the organization's settings and customizations.
In this example the invoice is also marked as paid and sent to the client's email using convenience properties send
and payments
, more details below.
Organization {id}
Replace the '{id}' in the URL with your created organization's ID.
"payments"
The payments
property contains payment information that will be added to the invoice, marking it as paid by a card. If the amount
field is not specified, the full invoice amount will be used. If you wish to create an invoice that has not been paid yet, simply remove this property. For more details about adding payments, see the Payments section.
"send"
By including the send
parameter, the invoice will also be sent automatically to the client's email using the default email message body set for the Organization when "send": true
is set. You can also define a custom message with each call by setting "send": { "message": "Custom message" }
, or by updating the Organization's Default invoice email message.
Want to download a PDF instead? Check the Download PDFs endpoint.
Read the full Document documentation >
Read the full Payment documentation >
const response = await fetch('https://api.spaceinvoices.com/v1/Organizations/{id}/Documents', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"type": "invoice",
"reference": "ORDER_NUMBER",
"_documentClient": {
"name": "Customer name",
"country": "United Kingdom",
"email": "EMAIL"
},
"_documentItems": [
{
"name": "Space suit",
"quantity": 1,
"price": 10000,
"_documentItemTaxes": [
{
"rate": 21
}
]
}
],
"payments": [
{
"type": "card"
}
],
"send": {
"message": "Thank you for your order."
}
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/Organizations/{id}/Documents' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"type": "invoice",
"reference": "ORDER_NUMBER",
"_documentClient": {
"name": "Customer name",
"country": "United Kingdom",
"email": "EMAIL"
},
"_documentItems": [
{
"name": "Space suit",
"quantity": 1,
"price": 10000,
"_documentItemTaxes": [
{
"rate": 21
}
]
}
],
"payments": [
{
"type": "card"
}
],
"send": {
"message": "Thank you for your order."
}
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/Organizations/{id}/Documents"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'type': 'invoice',
'reference': 'ORDER_NUMBER',
'_documentClient': {
'name': 'Customer name',
'country': 'United Kingdom',
'email': 'EMAIL'
},
'_documentItems': [
{
'name': 'Space suit',
'quantity': 1,
'price': 10000,
'_documentItemTaxes': [
{
'rate': 21
}
]
}
],
'payments': [
{
'type': 'card'
}
],
'send': {
'message': 'Thank you for your order.'
}
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.