The Space Invoices API handles all the necessary setup for processing stand-alone and invoice-based payments and collecting platform application fees for online payments. With our cloud-hosted UI, no additional development is required to enable this feature.
Our services include:
- Simple on-boarding for Organizations via our cloud-hosted UI
- A online invoice view page with an integrated "Pay now" button to collect payments directly on invoices
- Stand alone payment collection page
- Automated invoice status changes based on collected payments
- Automatic application fee collection with customization options
Enable Payment Processing
Enabling Payment Processing is a straightforward task within the Space Invoices platform. Here’s how to go about it:
- Begin by logging into your Space Invoices account at spaceinvoices.com.
- Once logged in, navigate to the
Payment Processing
section from the menu. - Within the
Payment Processing
section, locate theSpace Payments
box and click on theSet up
button.
Now, you'll need to provide some data for configuration:
Application Fee %
: Define the application fee percentage you aim to collect from Connect account charges.Payment Complete Return URL
(Optional): Specify a URL to which users will be redirected post the completion of invoice payments.
- Following this, a
Verification
window will appear on the same page. Click onOpen Form
to initiate the verification step.
Upon completing these steps, you're ready to advance to the next section: onboarding Organizations. This will further streamline your payment processing journey and enhance your invoicing capabilities.
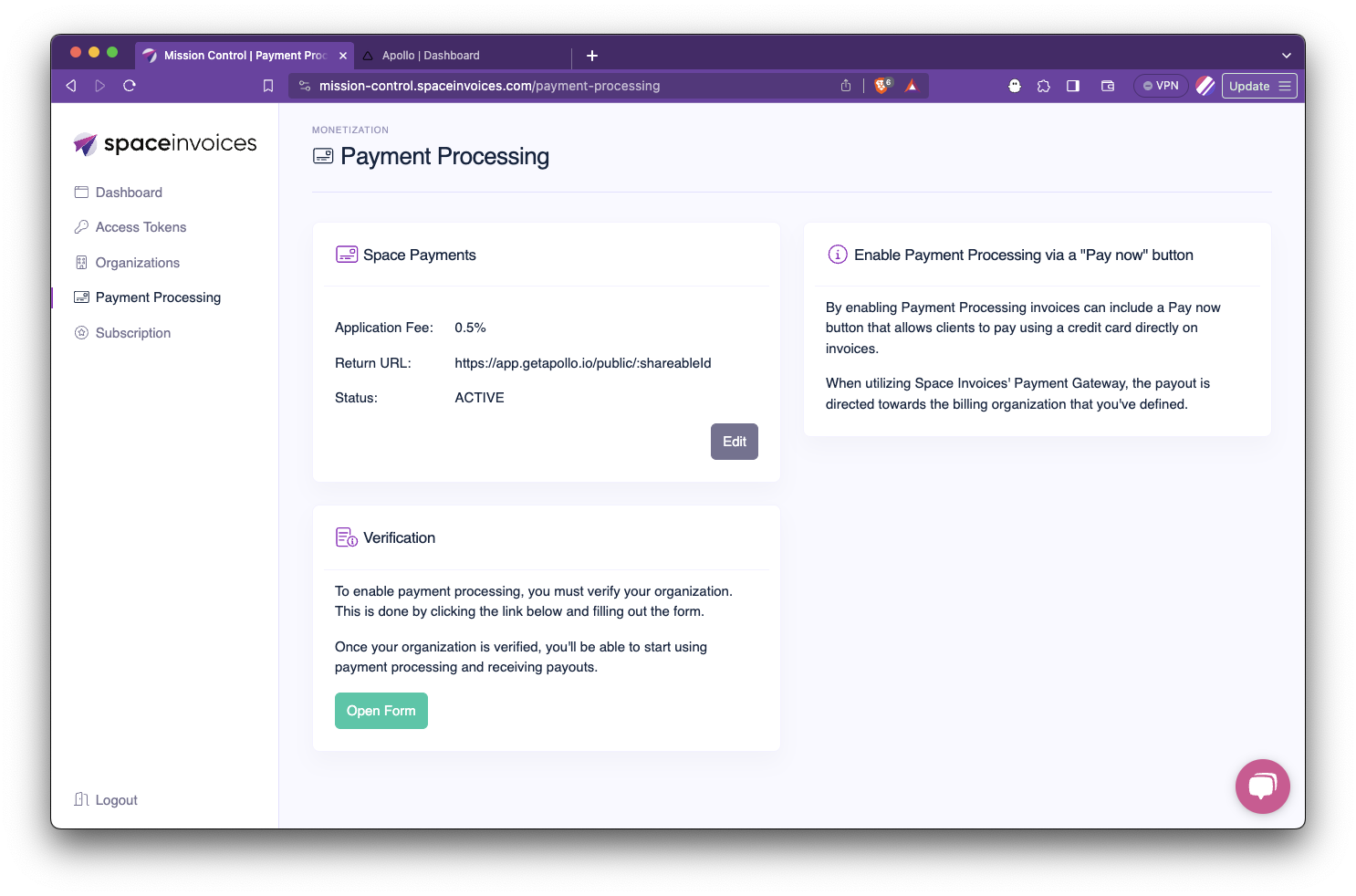
Onboard an Organization
Each Organization needs to be onboarded to enable collecting payments on invoices. We've made sure the process is as simple as possible for you and your users.
To initiate the onboarding process using our provided UI, direct your users to navigate to https://app.getapollo.io (or your provided white-label domain) and click on Settings > Payment Processing.
The Payment Processing menu item will only become available after Payment Processing has been enabled on your Space Invoices account (step 1 of this guide).
The user will be redirected to an onboarding page, where they will provide additional details about their organization required to complete the onboarding process.
Once an organization has been successfully onboarded and approved, the Payment Processing feature gets activated automatically. This means that a "Pay Now" button will now be visible on all issued invoices.
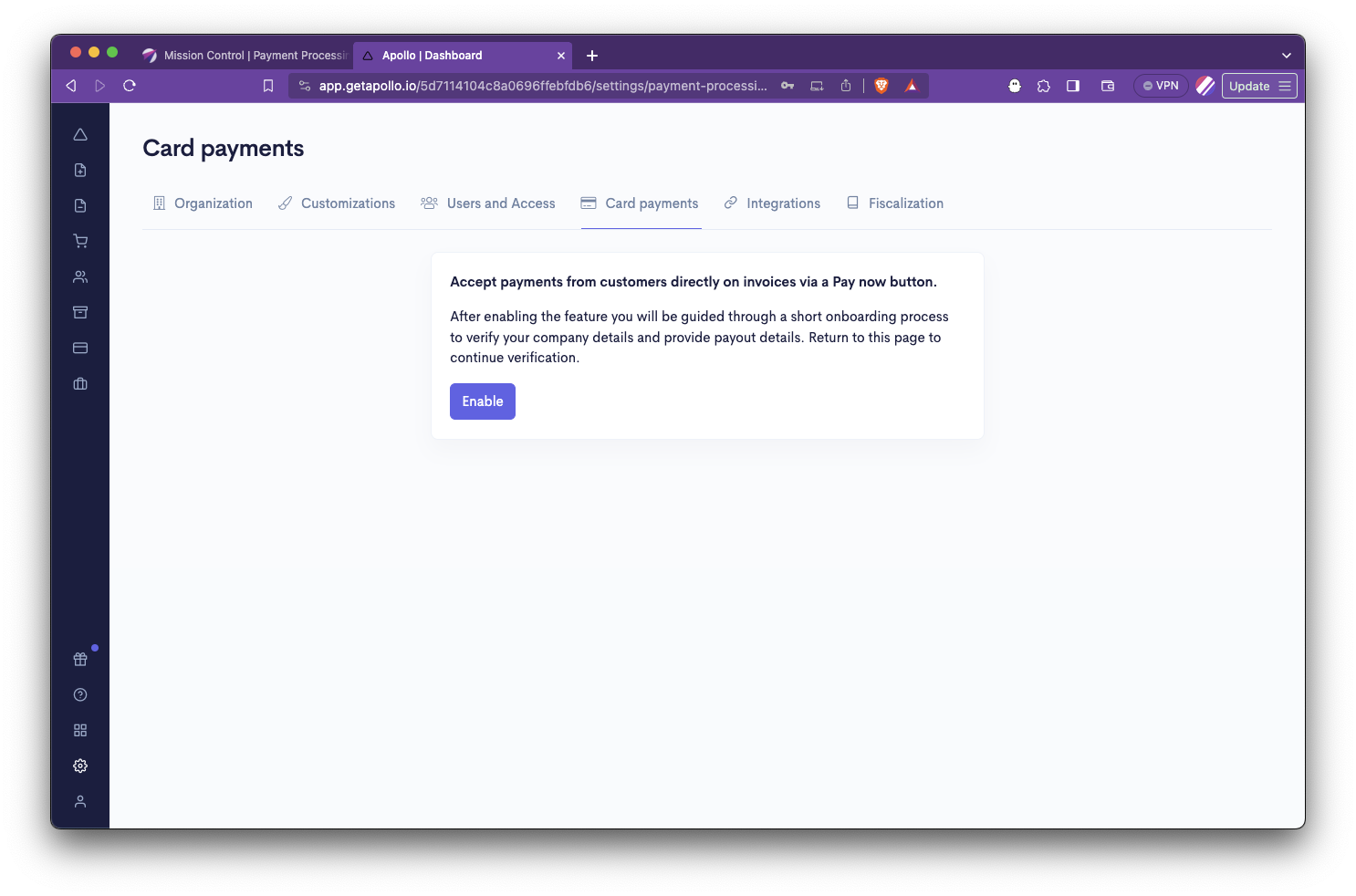
"Pay now" button
After the onboarding process is completed, any invoice shared with a client using the send endpoint will automatically include a "Pay" button and a checkout form for convenient payment processing.
Space Invoices takes care of automatically marking invoices as paid once the payment has been successfully processed. This ensures that your invoice management remains streamlined and up-to-date, allowing you to focus on other aspects of your business.
Create a Payment Intent
To create a custom checkout flow a Payment Intent can be created via the Space Invoices API.
"amount"
Amount to charge i.e. 100 for one hundred.
"currencyid"
(optional)
Currency to collect payment in. Automatically populated based on organization default currency.
"allowRecurring"
(optional)
Enables capturing consecutive payments after this payment without additional user confirmation.
The returned Payment Intent object will contain a link to the checkout page in the "paymentLink"
property.
const response = await fetch('https://api.spaceinvoices.com/v1/organizations/{id}/payment-intents', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"amount": 100,
"reference": "123456",
"returnURL": "https://example.com/payment-successful"
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/organizations/{id}/payment-intents' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"amount": 100,
"reference": "123456",
"returnURL": "https://example.com/payment-successful"
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/organizations/{id}/payment-intents"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'amount': 100,
'reference': '123456',
'returnURL': 'https://example.com/payment-successful'
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"amount": 100,
"reference": "123456",
"returnURL": "https://example.com/payment-successful",
"paymentLink": "https://pay.spaceinvoices.com/...",
"organizationId": "5f1d2c6b6ee48100249d5d7d",
"currencyId": "EUR",
"status": "initiated",
"expiresAt": "2023-04-28T19:41:00.930Z"
}
Create a recurring charge
To automatically charge a card based on a previously successful payment intent an authorized payment intent can be created.
"initialPaymentIntentId"
ID of previous successfully paid Payment Intent. The same ID can be used for multiple consecutive payments.
"amount"
Amount to charge i.e. 100 for one hundred.
"currencyid"
(optional)
Currency to collect payment in. Automatically populated based on organization default currency.
const response = await fetch('https://api.spaceinvoices.com/v1/organizations/{id}/payment-intents/authorized', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"initialPaymentIntentId": "644c214c6ee48100249d5d7c",
"amount": 100,
"reference": "123456"
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/organizations/{id}/payment-intents/authorized' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"initialPaymentIntentId": "644c214c6ee48100249d5d7c",
"amount": 100,
"reference": "123456"
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/organizations/{id}/payment-intents/authorized"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'initialPaymentIntentId': '644c214c6ee48100249d5d7c',
'amount': 100,
'reference': '123456'
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"amount": 100,
"reference": "123456",
"organizationId": "5f1d2c6b6ee48100249d5d7d",
"currencyId": "EUR",
"status": "captured",
"succeeded": true
}