The following instructions outline the APIs available for building your own Stripe Connect onboarding and payment flows while still leveraging the payment processing and invoice management capabilities provided by Space Invoices through Stripe Connect's API.
If you're looking for an express setup, pre-built public invoice and payment pages using our cloud-hosted UI please take a look at Stripe Connect section.
Enable Stripe Connect
To enable Stripe Connect on your Space Invoices account, you'll need to provide your Stripe API credentials and specify the desired application fee percentage.
We offer a user-friendly interface for enabling Stripe Connect on spaceinvoices.com. Simply log in to your Space Invoices account, and access the Payment Processing option from the main menu. From there, you can input your Stripe API credentials and set the application fee percentage according to your preferences.
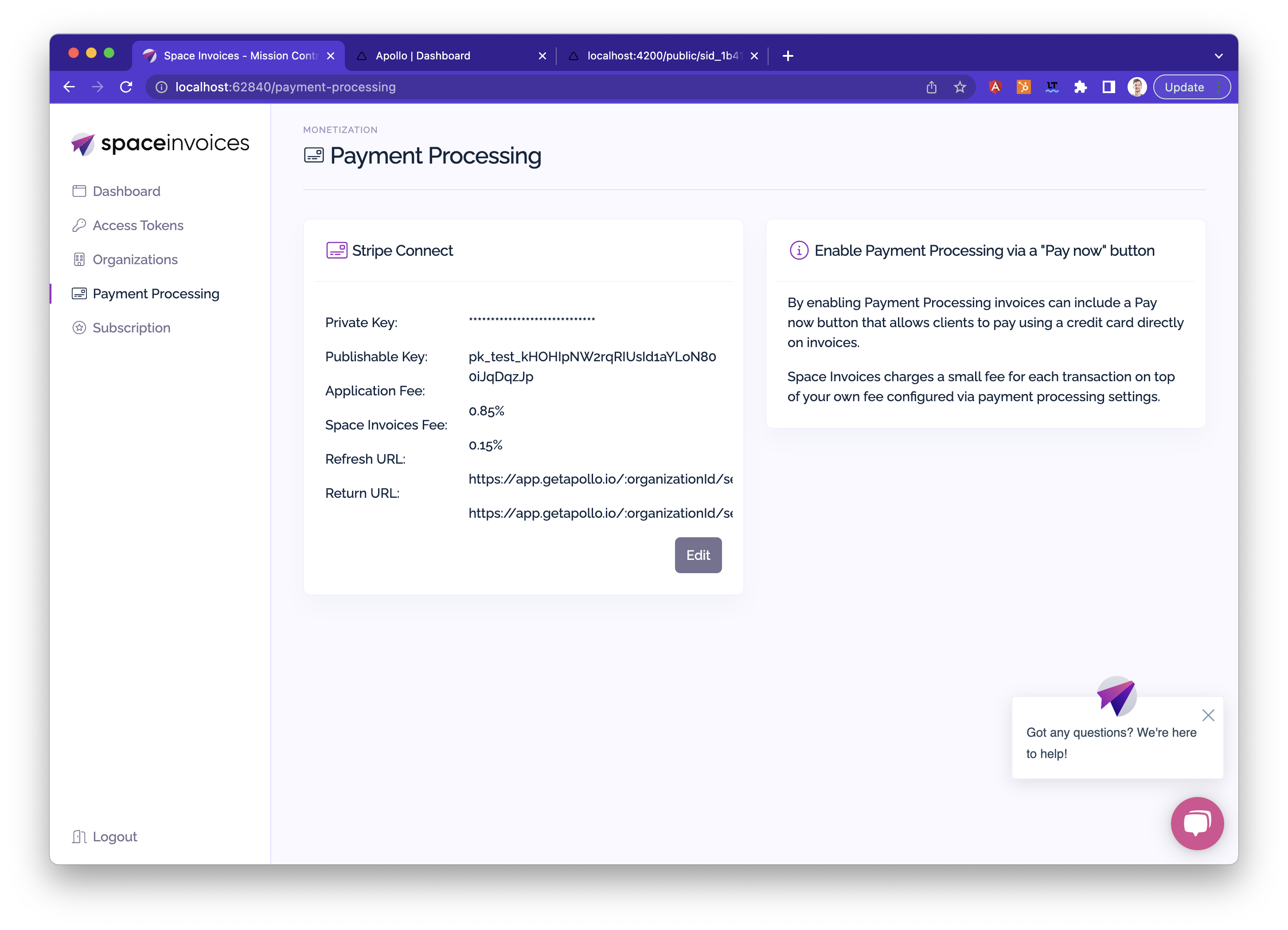
Enable Stripe Connect via API call (alternative)
Alternatively, Strepe Connect can also be enabled via API call as demonstrated on in the example.
const response = await fetch('https://api.spaceinvoices.com/v1/stripe-connect', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({
"privateKey": "pk_...",
"publicKey": "sk_...",
"applicationFee": 1,
"refreshUrl": "https://...",
"returnUrl": "https://..."
})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/stripe-connect' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{
"privateKey": "pk_...",
"publicKey": "sk_...",
"applicationFee": 1,
"refreshUrl": "https://...",
"returnUrl": "https://..."
}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/stripe-connect"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
'privateKey': 'pk_...',
'publicKey': 'sk_...',
'applicationFee': 1,
'refreshUrl': 'https://...',
'returnUrl': 'https://...'
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"privateKey": "pk_...",
"publicKey": "sk_...",
"applicationFee": 1,
"spaceInvoicesFee": 0.15,
"refreshUrl": "https://...",
"returnUrl": "https://...",
"accountId": "644c214c6ee48100249d5d7e",
"createdAt": "2023-04-28T19:41:00.930Z",
"updatedAt": "2023-04-28T19:41:00.930Z",
"custom": {}
}
Onboard an Organization
Each Organization needs to be onboarded to Stripe Connect to enable receiving payments on invoices.
The process includes two steps, 1 creating a Stripe Connect Account for the Organization, and 2 creating an on-boarding link where they can provide additional information required by Stripe. Step 2 is documented in the next section.
const response = await fetch('https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"active": true,
"chargesEnabled": true,
"stripeConnectId": "644c214c6ee48100249d5d7e",
"stripeAccountId": "644c214c6ee48100249d5d7f",
"organizationId": "644c214c6ee48100249d5d7a",
"createdAt": "2023-04-28T19:41:00.930Z",
"updatedAt": "2023-04-28T19:41:00.930Z",
"custom": {}
}
Check status of onboarding
This endpoint provides a way to check if Stripe Connect Account was created for an Organization and if on-boarding has been completed allowing payment processing to take place.
If the request returns a reposponse status 404
a Stripe Connect Account has not been initiated yet. See previous section to start the process.
The property chargesEnabled
in the response body indicates if on-boarding was successfull and payment processing is enabled. To complete on-boarding see the next section.
const response = await fetch('https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account', {
method: 'GET',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
}
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X GET 'https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
response = requests.get(url, params={}, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"url": "https://..."
}
Generate an onboarding link
To complete on-boarding users should be directed to an on-boarding page where they can provide additional details required by Stripe.
The user should be directed to the URL returned in the response body. The endpoint can be used multiple times to generate new URLs if on-boarding has not been completed yet.
To check the status of on-boarding see the previous section.
const response = await fetch('https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account/link', {
method: 'POST',
headers: {
"Authorization": "ACCESS_TOKEN",
"Content-Type": "application/json",
},
body: JSON.stringify({})
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X POST 'https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account/link' \
-H 'Authorization: ACCESS_TOKEN' \
-H 'Content-Type: application/json' \
-d '{}'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/organizations/{id}/stripe-connect/account/link"
headers = {
'Authorization': 'ACCESS_TOKEN',
'Content-Type': 'application/json'
}
payload = {
}
response = requests.post(url, json=payload, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"id": "644c214c6ee48100249d5d7d",
"active": true,
"chargesEnabled": true,
"stripeConnectId": "644c214c6ee48100249d5d7e",
"stripeAccountId": "644c214c6ee48100249d5d7f",
"organizationId": "644c214c6ee48100249d5d7a",
"createdAt": "2023-04-28T19:41:00.930Z",
"updatedAt": "2023-04-28T19:41:00.930Z",
"custom": {}
}
Custom "Pay now" button
To collect payments for individual invoices, you can use Stripe Checkout UI components in your user interface. For more information on how to use Stripe Checkout, refer to the Stripe Documentation.
The Stripe UI components require the publicKey
and clientSecret
values, which are returned in the response body of the following API request. Make this request whenever the checkout form is generated, ensuring that the correct data is passed to the Payment Intent in case the invoice is updated.
The returned Payment Intent is configured by the API with the correct amount due and currency, based on the invoice being paid. Once the payment is processed, Space Invoices automatically marks the invoice as paid.
The use of shareableId removes the need for authorization of the request which allows presenting a public invoice and checkout page to clients.
const response = await fetch('https://api.spaceinvoices.com/v1/Documents/{shareableId}/stripe-connect/payment-intent', {
method: 'GET',
headers: {
"Content-Type": "application/json",
}
});
Login to auto-populate your access token.
Your access token is displayed in examples.
Prerequisite: npm install node-fetch
curl -X GET 'https://api.spaceinvoices.com/v1/Documents/{shareableId}/stripe-connect/payment-intent' \
-H 'Content-Type: application/json'
Login to auto-insert your own access token.
Your access token displayed in examples.
import requests
url = "https://api.spaceinvoices.com/v1/Documents/{shareableId}/stripe-connect/payment-intent"
headers = { 'Content-Type': 'application/json'
}
response = requests.get(url, params={}, headers=headers)
Login to auto-insert your own access token.
Your access token displayed in examples.
Response:
{
"publicKey": "sk_...",
"clientSecret": "...",
"paymentIntentId": "...",
"amount": 1000
}